Cloning page elements with JavaScript
Use the cloneNode() method, and set its argument to 'true' if you also want to clone all of the element's child nodes.
Using Python in your HTML document
see here
Element manipulation with javascript
1. Rollovers
Method 1: Changing the source attribute of the image just using HTML. (Can cause lag)
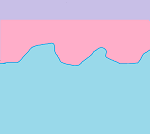
Method 2: Using JS to change the class
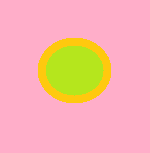
The code controlling the image's behaviour on rollover is in the index.js file.
Method 3: using a JS function to target all images of a class
These images are within link tags. The javascript file looks for all images whose parents tags are links tags, and applies a rollover function that swaps their source image.
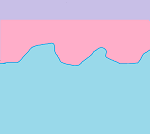
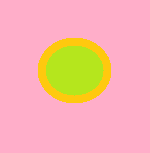
Element Manipulation with JQuery Events
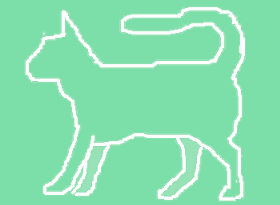
JQuery selectors are used to determine the behaviour of the buttons when clicked.
$(document).ready(function(){ $("#hide-button-1").click(function(){ $("#hide-element-1").hide()}) $("#show-button-1").click(function(){ $("#hide-element-1").show()}) })
Chapter 1: Making a blog from scratch
There is basic advice on this site: code.makeryThis page might be worth a read? site structure tips from the web style guide.
Trying out some 'CSS-Tricks' Tricks
Neon text
(See here) - Creates appearance of glowing neon text just using multiple layers, with increasing blur-radius, of the text-shadow property.
Flashy what
The text is animated using keyframes.
SVG Graphics and simple animations using CSS and Keyframes
CSS Specificity
For any page element there are almost always conflicting styles affecting how it should be displayed in the browser. The specificity rules exist to clarify to the browser which one should be dominant in each case.
Firstly, the very concept of cascading means that unless you specify otherwise, an element will automatically inherit the CSS styling of its parent. (This is called 'inheritance'.)
Also that, given styles that would otherwise be equal in specificity, more recent styles will take precedence. 'Most recent' means 'closest to the bottom of the sheet', or 'closest to where the element itself appears in the html'.
The situation can quickly become very complicated with the addition of classes, ids, and inline styles. An algorithm involving four style selector categories is used to work out which style should apply.
A few general notes on the hierarchy:
Inline <style> tags will override stylesheet styles for an element. !important after a property value can be used to over-ride any specificity rule or inline style. Eg color: green !important; But in general it should be avoided.
Selector specificity
Basically anything that identifies an element is a selector, eg:
- Types like Div, id, class, a
- pseudo-classes like :hover
- pseudo-classes like :first-child, first-of-type
How it works:
Selector specificity = 0, 0, 0, 0
Consider it is a number without the commas: eg 0000 < 0100 < 1010 The higher the value of this number is, the more specific it is.
Child versus Descendant
Aside Note on CSS Efficiency
Note: For the sake of decreasing the browser's workload, it's better to use shorter selectors wherever possible. This means reducing the amount of child and descendant selectors.
Some Preliminary notes on JavaScript
About jQuery: link to some jQuery methods. This is maybe useful? (About blog navigation)
Including javascript in your html file: you should encourage the webpage to load the javascript LAST by linking to the script near the end of the document, most commonly just before the </body> tag. Otherwise it might have awkward results as loading of the javascript file slows down the rest of the page.
<script type="text/javascript" src="somefile.js"></script>
Git Commands
Configuring your remote repository
This command will show you the names of your remote repos. ('Origin' will appear as the name of the default server that you cloned your local repo from.)
cloning a remote repo to your local hostTo push to a specific branch:
Making your current branch the default branch:
"You can set a global option for “git push” to push the code to the current branch by executing the following command:git config --global push.default currentNow you can just type…git push
Source: https://hayato-iriumi.net/2020/10/13/git-push-to-the-current-branch/
Merging changes you've made on a branch into the master
First you need to change back to the master, by checking it out:
Now you have a local copy of the master. You are going to add the changes to the local master first, with the command:
git merge branch-name
then you push the updated and merged master back to the remote repo:
Changing or updating a remote repository location
If you change the name of your repository on GitHub, git will still redirect your commits to the new location, but you should update it anyway, in case someone else starts using the old repository name (then the redirect will no longer happen)
For addresses using an SSH key:
For HTTPS:// addresses:
Responsive Design
Read these articles:
- Site Wizard - very useful section on including media query in stylesheet links in head.
- Screen sizes and responsivity test (also has a good explanation of breakpoints)
- list of device screen sizes and tips on how to use breakpoints
- even better breakpoint explanation
Putting the following tag in the doc's head over-rides the mobile browser's trick of "pretending" to have a bigger screen than it actually does, and prevents it squashing all the content to fit:
Note: "Friction" in web design terms basically just means unnecessary clutter or steps to complete an action on a website, or slow-loading elements that make the experience frustrating.
Page Layout with HTML and CSS
"An important concept to understand first is that every single element on a web page is a block. Literally a rectangle of pixels."
(CSS Tricks)
A bit of layout history: page layouts were initially constructed using the html <table> tags to distribute content in rows and colomns. Then CSS enabled the positioning and float properties. More recently, Flex Box and Flex properties allow docs to flow and scale much more flexibly. Grid layout is the most recent development (and not yet fully supported).
Using the Position property:
"Static"is the default value, and means for an element to sit wherever it would usually sit in the HTML.
"Absolute" positioning instead fixes an element outside the normal HTML flow. It is usually combined
with properties that specify its exact position on the page, namely:
Top
Right
Bottom
Left
(You only specify one property for vertical and one for horizontal, if needed at all).
The abolute-positioned element will be placed relative to the <body> element, or to the "closest"
non-statically-positioned element.
It is designated in pixels relative to either the nearest absolute-postioned parent element, of the HTML page itself (the root block, or "initial containing element"), if no such element is found.
Relative positioning is actually basically the same as absolute positioning, except that it takes as its reference where it would have been positioned if in normal flow.
Relative position of an element means relative to its static position, and defining the position this way allows you to move the element, eg, 10px left or right from where it would otherwise sit.
See here for more detail: CSS-Tricks on positioning
Using BEM – Block Element Modifiers
...and naming conventions in CSS.
For CSS names, use hyphenated all-lowercase forms. Use a name that makes it obvious what a component is about. Then for all the elements that are part of this component, use the convention of two underscores and the element name.
Naming convention for elements:
.the-component__part {}
the elements are child components of the parent.
Naming convention for modifiers:
.the-component--red {}
two hyphens (here the component is modified)
.the-component__part--red
here the element 'part' is being modified.
About JSX
JSX is a weird html-JavaScript-looking hybrid that is used as a go-between for JavaScript and React. It makes using React easier as it firstly makes the structure more readable by being written in familiar-looking html, and and secondly, allows you to define things called 'Components' that are easy to reuse, instead of typing out a lot of React JavaScript code repeatedly.
The JSX is included in the .js file amongst your other JavaScript. A Transpiler called Babel will transpile the JSX into JavaScript for the browser - the include the src link to Babel in the head of the .html file.
<script src="https://unpkg.com/@babel/standalone/babel.min.js"></script>